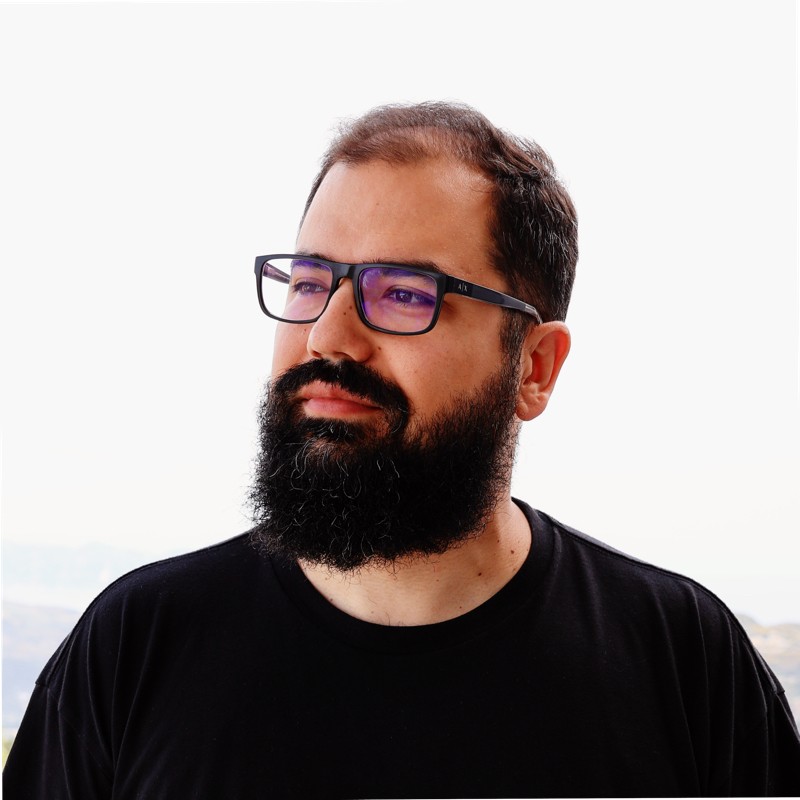
Navigating state management in 2024
May 08, 2024
State Management in 2024: Navigating the Complex Landscape
In the ever-evolving world of software development, state management remains a critical, yet challenging aspect. As we navigate through 2024, the landscape of state management has expanded, introducing new paradigms, tools, and methodologies that promise to simplify the complexities involved in managing state in modern applications. This article aims to dissect these advancements, offering experienced developers a comprehensive guide to understanding and leveraging the latest in state management technologies.
The Evolution of State Management
State management has come a long way from the simple global variables and the rudimentary event-based systems of the early 2000s. Today, it encompasses a broad spectrum of strategies and tools designed to manage the state of applications across different platforms and architectures seamlessly.
The Rise of Reactive State Management
One of the most significant shifts we’ve seen is the move towards reactive state management. Libraries such as RxJS and frameworks like Vue 3 and Svelte have embraced this paradigm, offering developers a more intuitive way to handle data flows and state changes in their applications. Reactive state management allows for a more declarative approach to handling state, where changes propagate through the system automatically, reducing the boilerplate code and potential for errors.
// Example of reactive state management in Svelte
<script>
import { writable } from 'svelte/store';
const count = writable(0);
function increment() {
count.update(n => n + 1);
}
</script>
<button on:click={increment}>Increment</button>
<p>{$count}</p>
The Emergence of Global State Management Solutions
With Redux falling out of favor due to its verbosity and complexity, the community has shifted towards lighter, more intuitive solutions like Zustand and Recoil, which offer a more modern approach to global state management. These libraries provide a simpler API, better performance, and are more aligned with the React hooks paradigm.
// Example of global state management with Zustand
import create from 'zustand';
const useStore = create(set => ({
count: 0,
increment: () => set(state => ({ count: state.count + 1 }))
}));
function Counter() {
const { count, increment } = useStore();
return (
<div>
<button onClick={increment}>Increment</button>
<p>{count}</p>
</div>
);
}
State Management in Microfrontend Architectures
The adoption of microfrontend architectures has introduced new challenges and solutions in state management. Sharing state between independently deployable frontend units requires a careful approach to ensure consistency and maintainability.
Server-Side State Management
The boundaries between client and server have blurred with the advent of technologies like Next.js and Nuxt.js, which offer hybrid rendering capabilities. This shift has necessitated a reevaluation of state management strategies to encompass server-side considerations.
// Example of server-side state management in Next.js
import { useState } from 'react';
export default function Home({ initialCount }) {
const [count, setCount] = useState(initialCount);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export async function getServerSideProps() {
// Fetch initial count from an API
const res = await fetch('https://api.example.com/count');
const initialCount = await res.json();
return { props: { initialCount } };
}
The Role of AI in State Management
An exciting development in 2024 has been the integration of AI and machine learning into state management. AI-driven tools are now capable of predicting state changes, optimizing state synchronization, and even automatically managing state based on user behavior and application performance metrics.
Best Practices for State Management in 2024
With the plethora of tools and methodologies available, it’s essential to adhere to best practices to ensure efficient and maintainable state management:
- Choose the Right Tool for the Job: Evaluate the needs of your application and choose a state management solution that aligns with its architecture and complexity.
- Embrace Immutability: Immutable state makes your application easier to debug, test, and reason about.
- Leverage Modularization: Break down your application state into manageable chunks to enhance maintainability and reusability.
- Optimize for Performance: Be mindful of the performance implications of your state management choices, especially in large-scale applications.
Conclusion
The landscape of state management in 2024 is rich and diverse, offering developers a wide range of tools and methodologies to choose from. By understanding the latest trends and best practices, experienced developers can navigate this landscape effectively, creating robust, scalable, and maintainable applications. As we look towards the future, the continued evolution of state management technologies promises to make managing application state an even more seamless and intuitive process.
State management is a journey, not a destination. As technologies evolve and new challenges arise, staying informed and adaptable is key to mastering state management in the modern era of software development.
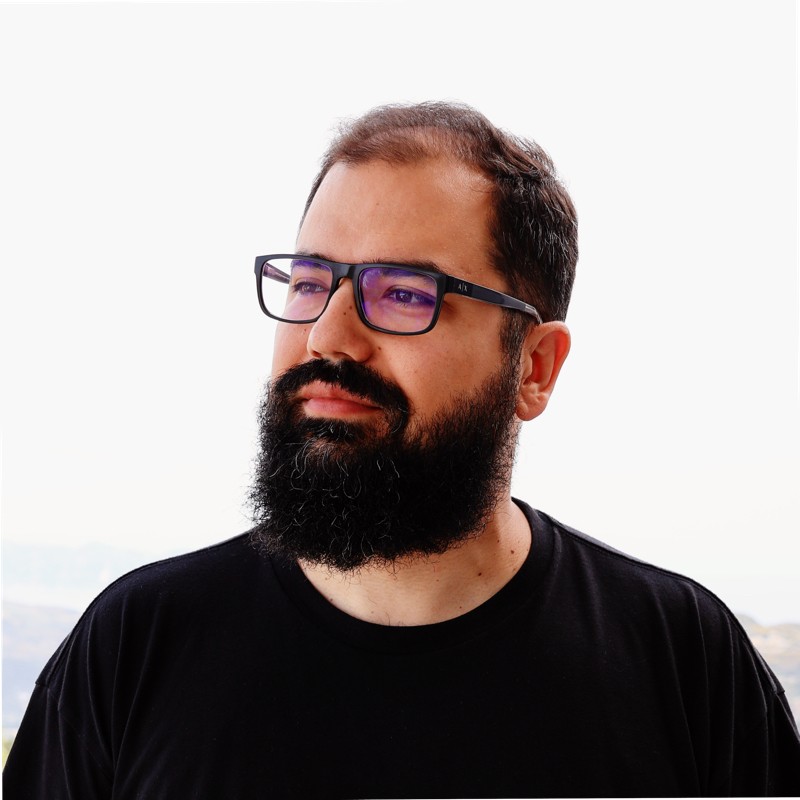